As developers, we’re expected to know a lot of random things. Sure, you can learn data structures and algorithms from a CS class, and you can learn frameworks from online tutorials or a bootcamp, but what about the other things? How to be more effective on the command line1? How to increase productivity on your computer? How to find things quickly in code? There’s no class for those.
I’m here to try and help. These are the tools, tips and advice I wish I had internalized when I was just starting out. Many of the details below are specific to macOS, but similar tips and tricks apply on other systems.
I’ve broken it down very roughly into the following categories:
1. Computer Setup
Turn your caps lock key into your control key
This sounds small, but it’s a big workflow upgrade. The control key is used all the time, but on a mac it’s in a difficult-to-hit position underneath and behind your pinky. The caps lock key, on the other hand, is a big, beautiful key in some seriously high-value real estate on your keyboard. Wouldn’t it be nice to swap them?
Go to System Preferences > Keyboard > Customize Modifier Keys
and turn caps lock into control:
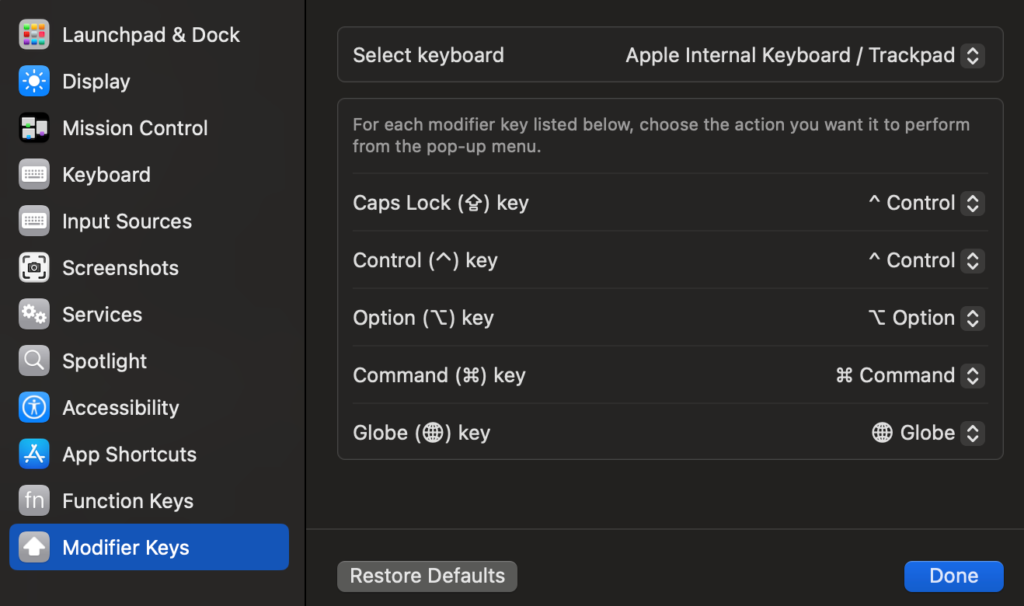
I have almost never needed my caps lock key2. I need my control key dozens of times every day.
Increase your key repeat speed
This is another small change that I love. The default mac key repeat speed is pretty slow, which is painful if you spend a lot of time in the terminal. There are shortcut commands to make your life easier, but it’s also nice to have faster cursor speed moving left and right.
Go to System Settings > Keyboard
and adjust your key repeat settings. This is what mine look like:
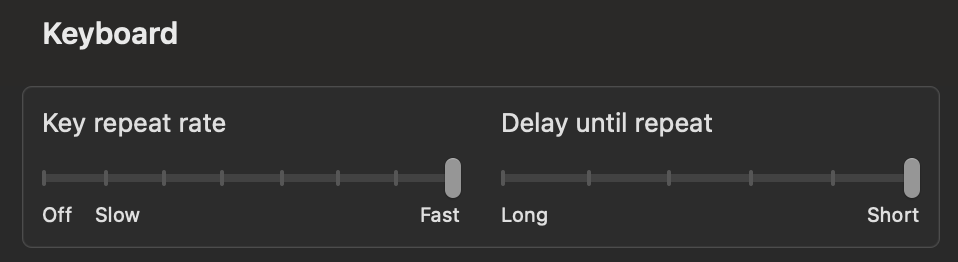
You may have to disable press and hold for accented characters to get the full benefit here, but I find the tradeoff worth it, and there are other ways to get those characters.
Get a window manager
Arranging multiple open applications across multiple monitors is a pain without a window manager. I used Spectacle for years until it was no longer maintained. Now I use Rectangle. There are others like Moom and Magnet which cost a few bucks. I don’t have strong feelings about which window manager to use, but I do have strong feelings that you should definitely use one.
2. Command-Line-Related Things
pbcopy and pbpaste
These give you command line access to your computer’s clipboard:
# Copy the contents of a file to your clipboard
cat payload.json | pbcopy
# Then go over to Slack and send it to your teammate
Or
# First, copy something to your clipboard from elsewhere
# Then paste it directly into a new file
pbpaste > payload.json
The !!
command
On the command line, !!
means re-enter the previous command
. Did you enter a long command which failed because it required sudo
powers? Just sudo !!
.
Use -
with cd
or git checkout
All devs know how to use cd
. But many don’t know that cd -
is short for “cd into the previous directory”.
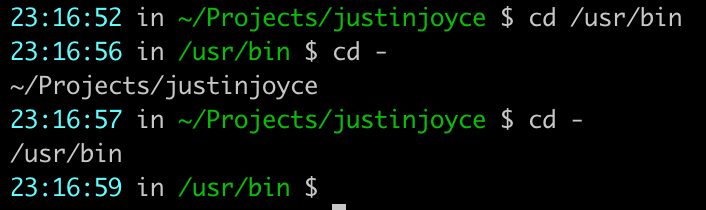
This tip also works when switching back and forth between git branches:

Count lines with wc -l
See a file but aren’t sure you want to open it because it might be huge?
# Do a quick sanity check
wc -l filename
Want to know how many files in your codebase contain random_thing
?
# How many files does "random_thing" appear in?
rg random_thing -l | wc -l
rg is ripgrep, discussed belowMake aliases for common commands
These can be for simple things like changing directories, or more complex things like tunneling into a remote machine with specific flags. Here are some of the aliases I use:
# Make it easy to get to my projects dir
alias pj="cd ~/Projects"
# list directories only
alias lsd="ls -dl ./*"
# Connect to a local pg instance on a non-standard port
alias local-db="psql -U postgres -h localhost -p 1123"
You can add aliases directly to your .bashrc
or .zshrc
, or you can add them to their own file and just source them from your rc
file like so: source ~/.aliases;
Take some time to set up your prompt
In the screenshots in this post, you can see what my terminal prompt looks like:

This is what works for me. Timestamp, current directory, git branch if I’m inside a repository, a %
flag for “uncommitted changes”, and a trailing $
to indicate that input starts there.
This prompt took me a while to settle on, and it took many hours of tinkering to get it just right. It is currently perfect for me; it clearly presents all the information I want. You should spend as much time as necessary to configure your prompt to your own taste.
Learn grep and then replace grep with ripgrep
Being able to find things in code is a foundational skill of being a developer, and grep
is the simplest way to do it. Native grep, however, is a bit slow. Fortunately, we have alternatives like the silver searcher and ripgrep. I use ripgrep
for its ridiculous, blazing speed, and I love it so much I wrote an entire post about it.
Write your local command history to log files
I have a full post devoted to this topic also! This tip has saved me countless hours of searching for “exactly the {complex_command_with_multiple_flags} I ran that one time 6 weeks ago to do {important_but_uncommon_task}”. I just go into my ~/.logs
directory and rg {some part of the command}
.
Learn command line navigation helpers…
Jumping around the command line can be slow and annoying. Increasing your key repeat speed helps, but there are better ways. These are a few I use often (notice they all use the control key):
control a
– jump to the beginning of inputcontrol e
– jump to the end of the inputcontrol u
– clear the entire line, empties the inputcontrol w
– delete back to the most recent word boundary (roughly)
These commands also work in interactive shells like the node console or IPython.
… Or navigate the command line with Vim keybindings
I got this tip from a comment on my post on Hackernews. By default, zsh and bash use Emacs keybindings—the navigation helpers in the previous section. But you can set your terminal to use Vim keybindings instead. I set this up as soon as I learned about it, and I like it so far.
Use Control d (yet another use of control
)
Most developers know that control c
will interrupt a running command. Surprisingly many devs don’t know that control d
can be used as an exit
command. If you’re in a psql
shell, or an interactive console, or even if you just want to close a terminal tab, you can hit control d
.
3. Technical but non-CS advice
Learn Regex
Regex3 is powerful and supported by virtually every language, with very minor differences in features. You don’t have to become a Regex expert, but learning the basics will serve you well for the rest of your career no matter what language / framework / tool you’re working in; you’ll be surprised how often it comes in handy.
Learn Vim
I know, you’ve heard this one before, but there’s a reason why Vim users are such proselytizers. I’m not advocating for becoming a hardcore, only-codes-in-raw-Vim devotee-people use IDEs for good reason-but I do think everyone should at least get familiar with Vim keybindings.
Learning Vim will be annoying, and it will slow you down significantly for a few weeks while you get up to speed. But if you stick with it, it will greatly accelerate your work forever after.
Plus Vim, like Regex, is available everywhere.
4. Potpourri
Screenshots
If you’re in a product engineering role, you’ll need screenshots. Here are a few options:
cmd shift 3
– screenshot your entire screen (or screens)cmd shift 4
– turns your cursor into a click and drag screenshot toolcmd shift 4
and then tapspacebar
– takes a screenshot of a single application window
Hold down control
while taking any screenshot to copy it directly to your clipboard instead of saving it to a file.
Find a good IDE
IDEs are great, and there’s a good chance your company will pay for a license for something like JetBrains. If they do, you should take them up on it. Good IDEs are packed with features that will make your life much easier, like running a single test case within a test file via a keyboard shortcut (control shift r
for JetBrains). I sometimes load up a repository in PyCharm or GoLand just so they can index the code and I can click my way through some definitions.
If you don’t want to try something heavier like PyCharm, or your company won’t cover it4, VS Code is free and very good for most everything, especially Typescript. I use both VS Code and JetBrains IDEs daily, depending on the task.
Command Line Manual Pages
Curious about a command? Read its manual page. man ls
will give you more information than you ever wanted to know about the ls
command.
Manual pages overwhelming? Check out tldr for the highlights.
Consider what currently annoys you
Do you wish you could more easily view your changes and commit small pieces? Take a look at tig. Do you connect to lots of different postgres instances? Maybe you would benefit from setting up a pgpass file. Do you work with kubernetes and constantly forget the right kubectl
commands? Maybe k9s would help.
Odds are if a task is bothering you it has also bothered another engineer before you, and that engineer might have built a tool to help.
Examine your existing tools
Do you know what t
does in github? Press it. What about cmd k
? What if you just enter a question mark? Did you know that Gmail supports some Vim keybindings? Do you know your editor’s shortcut for find all usages of this function
? Have you tried using console.group when debugging javascript?
Lots of our tools (especially IDEs) have very helpful built-in shortcuts and helpers, you just have to do a bit of digging to find them.
Conclusion
That’s it for now, but I’m constantly learning new tips and tools5, and I’ll keep coming back and updating this post as I do.
I hope you found this useful.
Notes
- This post was inspired by another post I read recently: Advanced macOS Command-Line Tools. ↩︎
- If you really need caps lock, just learn Vim and upcase to your heart’s content. ↩︎
- Regex is a deep topic, I’m working on a separate post devoted to it. ↩︎
- JetBrains really nailed the “too expensive for me to buy” but “not expensive enough that my company is likely to care” pricing model. ↩︎
- I’ve been working with Kubernetes for years and only just learned about K9s last week, but I’m already hooked. ↩︎