Git stash is for stashing away changes you need but aren’t quite ready to commit.
The command saves your local modifications away and reverts the working directory to match the HEAD commit.
– Git Docs
There are a few important stash-related commands.
Git stash
Say you’re on the main
branch, and you started making edits before checking out a new branch for development:
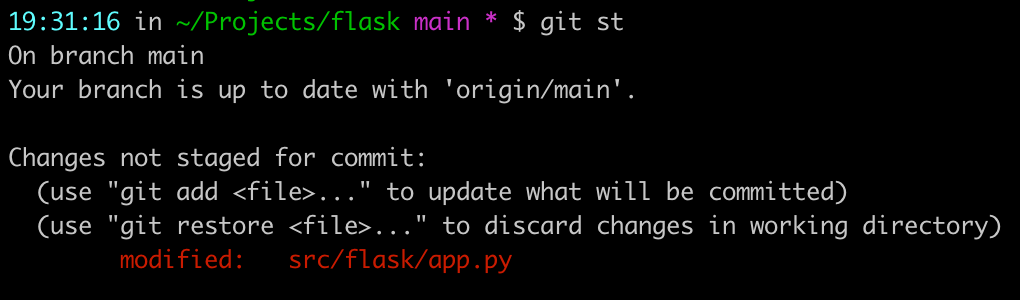
main
Making changes directly to main
is a no-no, so let’s move them to a new branch via:
git stash
git checkout
a new branchgit stash pop
to put the stashed code back
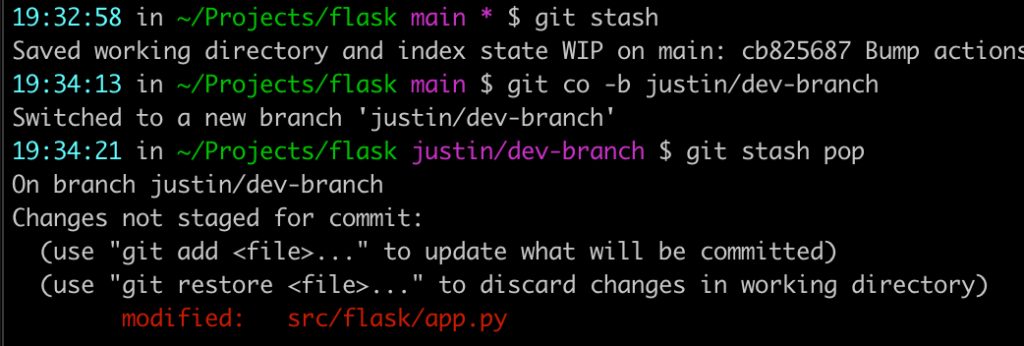
Git stash branch
This is a lesser-known alternative to the three steps above. Git stash branch takes the code from a stash entry and applies it to a brand new branch.
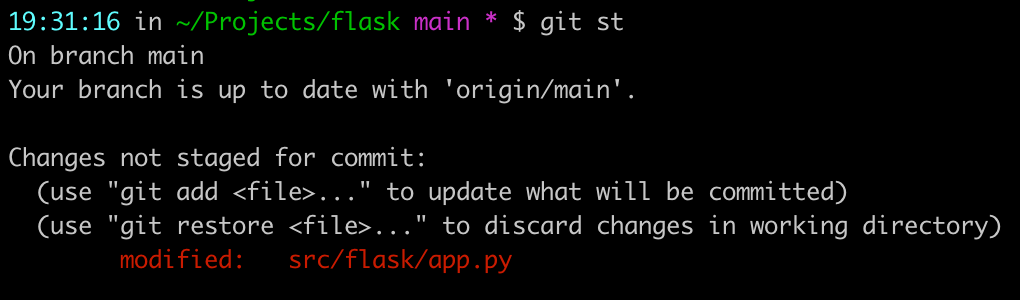
main
againI can put that code directly onto a new branch like this: git stash branch <new branch name>
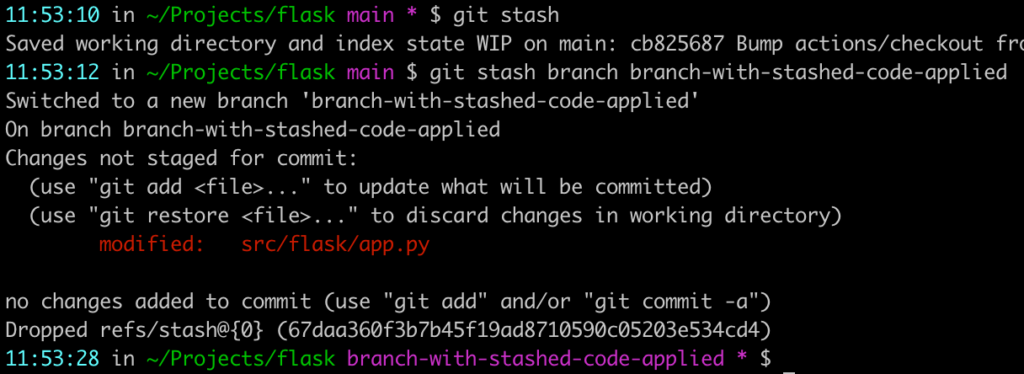
git stash branch
created a fresh branch and applied my stashGit stash list
This will list all of your existing stashes, with a leading identifier formatted like stash@{number}
:
$ git stash list
stash@{0}: WIP on branch-with-stashed-code-applied: cb825687 Bump actions/checkout from 3.5.2 to 3.5.3 (#5186)
stash@{1}: WIP on branch-with-stashed-code-applied: cb825687 Bump actions/checkout from 3.5.2 to 3.5.3 (#5186)
stash@{2}: On main: enables port forwarding to staging services
Above are three stash entries for this repository, each labeled with its source branch and a message. By default, the stash message is just the most recent commit message on that branch. But you can pass a custom stash message via git stash save "message"
.
Git stash save
This works exactly the same as git stash
, except that you can provide a helpful description for yourself for later e.g git stash save {description}
:
# Save a stash with a specific message
git stash save "enables port forwarding to staging services"
This comes in handy for things like local development hacks which you don’t want to commit, but might need again in the future. You can keep them saved, and then git stash apply
whenever you need the functionality.
Git stash apply
This applies a stash entry without removing it from your stash list. Using the same list from earlier:
$ git stash list
stash@{0}: WIP on branch-with-stashed-code-applied: cb825687 Bump actions/checkout from 3.5.2 to 3.5.3 (#5186)
stash@{1}: WIP on branch-with-stashed-code-applied: cb825687 Bump actions/checkout from 3.5.2 to 3.5.3 (#5186)
stash@{2}: On main: enables port forwarding to staging services
We can run git stash apply
to apply some stashed code:
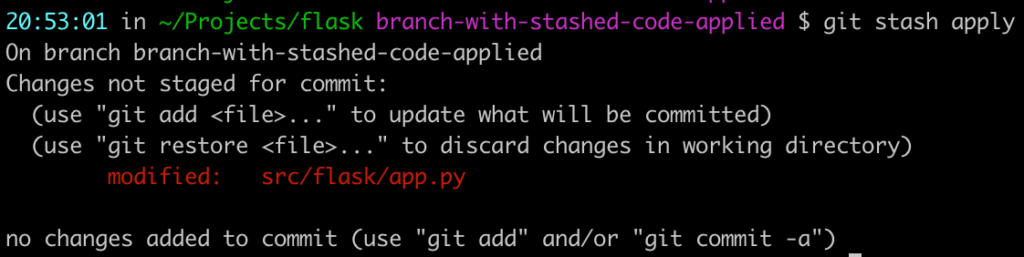
By default, it will apply the first entry in your stash list (stash@{0}
). To use a specific stash entry, pass its identifier via git stash apply stash@{number}
. The changes from stash@{number}
will be added to your branch, and stash@{number}
will remain in your stash list for use another time.
Git stash pop
This works like git stash apply
, but it removes the stash entry from your stash list after applying it.
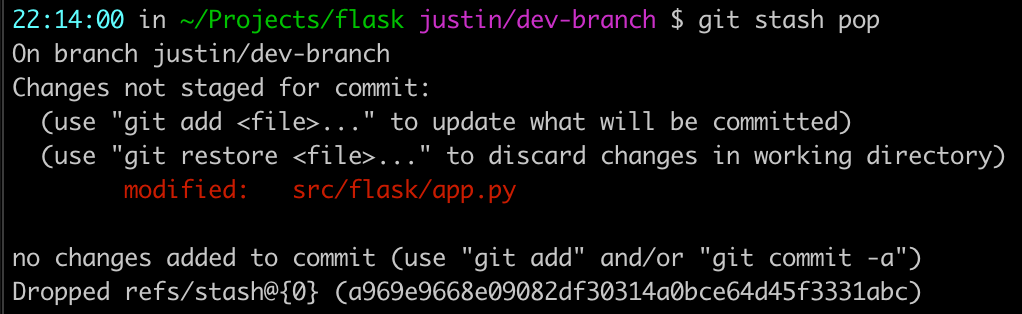
refs/stash@{0}
Now when I git stash list
again, I’m down to two entries instead of three:
$ git stash list
stash@{0}: WIP on branch-with-stashed-code-applied: cb825687 Bump actions/checkout from 3.5.2 to 3.5.3 (#5186)
stash@{1}: On main: enables port forwarding to staging services
Like apply, pop
defaults to the most recent stash entry, but you can pass in a specific stash id here too.
Git stash drop
Use git stash drop
to remove an entry from your stash list without applying its code, it will be deleted. The API is the same as apply
and pop
:
$ git stash drop stash@{1}
Dropped stash@{1} (b521d36c90d33cb36228f890ab6d9b831dfcb117)
Now I’m down to a single stash entry:
$ git stash list
stash@{0}: On main: enables port forwarding to staging services
Git stash show
Had a stash sitting on a branch for a long time and not sure exactly what it does? You can use git stash show
for a quick overview:
$ git stash show
src/flask/app.py | 3 +++
1 file changed, 3 insertions(+)
Then you can decide whether you want to leave it, apply it, or drop it altogether.
Git stash clear
Git stash clear removes all of your stash entries for a repository; it’s like running a bulk git stash drop
. It’s useful if you’ve accumulated lots of stash entries over a long time period, but it can be dangerous. Once you run git stash clear
, any stashed code is gone for good. The official git docs contain a warning that cleared stashes “may be impossible to recover”, so double check your stashes before you run this one.
Those are the basics! For a lot more detail, check out the official git docs for stash.